Edifice#
Declarative GUI framework for Python and Qt
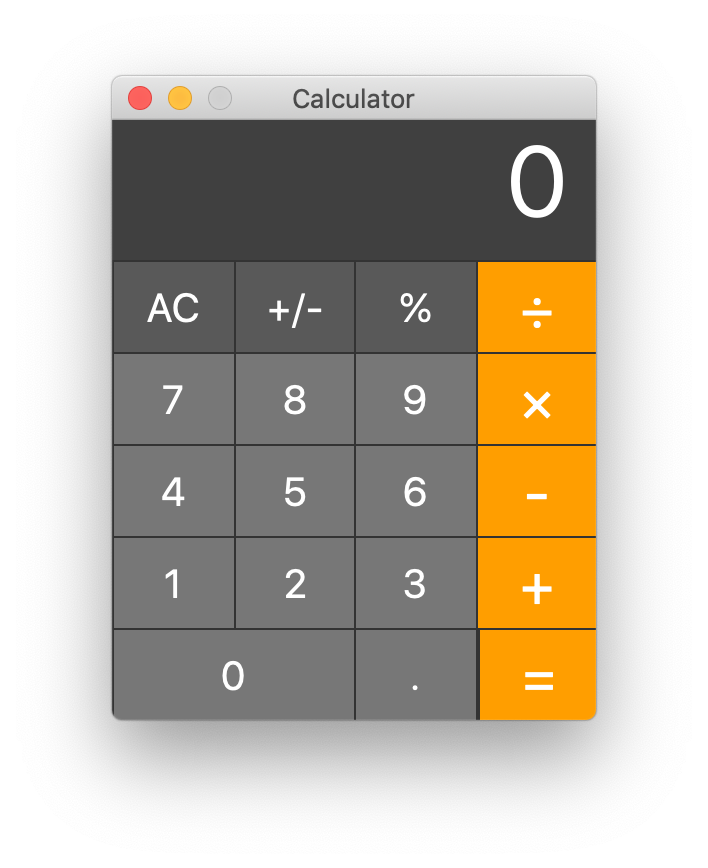
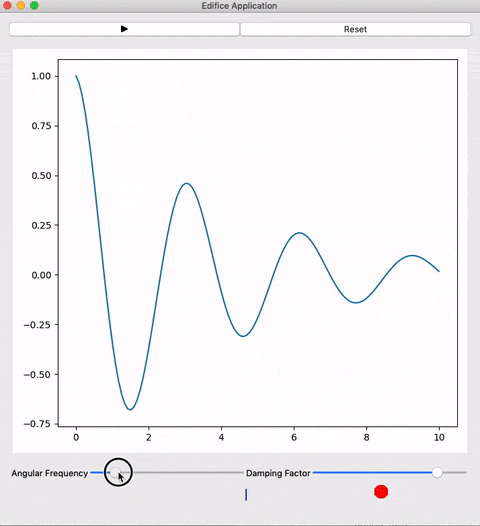
pip install PySide6
pip install pyedifice
from edifice import App, Window, Label, component
@component
def HelloWorld(self):
with Window():
Label("Hello World!")
App(HelloWorld()).start()
Edifice is a Python library for building declarative application user interfaces.
Modern declarative UI paradigm from web development.
100% Python application development, no language inter-op.
A native desktop app instead of a bundled web browser.
Fast iteration via hot-reloading.
This modern declarative UI paradigm is also known as “The Elm Architecture,” or “Model-View-Update.”
Edifice uses PySide6 or PyQt6 as a backend. Edifice is like React, but with Python instead of JavaScript, and Qt Widgets instead of the HTML DOM. If you have experience with React, you will find Edifice very easy to pick up.
Getting Started#
The easiest way to get started is via the Tutorial. To understand the core conception behind Edifice, see Edifice Core.
Why Edifice?#
Declarative
The premise of Edifice is that GUI designers should only need to worry about what is rendered on the screen, not how the content is rendered.
Most existing GUI libraries in Python, such as Tkinter and Qt, operate imperatively. To create a dynamic application using these libraries, you must not only think about what to display to the user given state changes, but also how to issue the commands to achieve the desired display.
Edifice allows you to declare what should be rendered given the current state, leaving the how to the library.
User interactions update the state, and state changes update the GUI. You only need to specify what is to be displayed given the current state and how user interactions modify this state.
With Edifice you write code like:
number, set_number = use_state(0)
with View():
Button("Add 5", on_click=lambda event: set_number(number+5))
Label(str(number))
and get the expected result: the GUI always displays
a button and a label displaying the current value of number
.
Clicking the button adds 5 to the number
,
and Edifice will handle updating the GUI.
Developer Tools
Declarative UIs are also easier for developer tools to work with. Edifice provides two key features to make development easier:
Dynamic hot-reloading of changed source code.
Element inspector.
See Developer Tools for more details.
Edifice vs. QML
QML is Qt’s declarative GUI framework for Qt. Edifice differs from QML in these aspects:
Edifice programs are written purely in Python, whereas QML programs are written in Python + a special QML language + JavaScript.
Because Edifice interfaces are built in Python code, binding the code to the declared UI is much more straightforward.
Edifice makes it easy to create dynamic applications. It’s easy to create, shuffle, and destroy widgets because the interface is written in Python code. QML assumes a much more static interface.
An analogy is, QML is like HTML + JavaScript, whereas Edifice is like React.js. While QML and HTML are both declarative UI frameworks, they require imperative logic to add dynamism. Edifice and React allow fully dynamic applications to be specified declaratively.
Extendable
Edifice is still currently under development, and some Qt widgets/functionality are not supported. However, it is easy to interface with Qt, either incorporating a Qt Widget into an Edifice component, use Qt commands directly with an existing Edifice component, or incorporating Edifice components in a Qt application.
Table of Contents#
- Tutorial
- Examples
- Edifice Core
- Base Elements
- edifice.QtWidgetElement
- edifice.Window
- edifice.ExportList
- edifice.View
- edifice.ScrollView
- edifice.TabView
- edifice.GridView
- edifice.TableGridView
- edifice.FlowView
- edifice.Label
- edifice.Image
- edifice.ImageSvg
- edifice.Icon
- edifice.IconButton
- edifice.Button
- edifice.ButtonView
- edifice.TextInput
- edifice.SpinInput
- edifice.CheckBox
- edifice.RadioButton
- edifice.Slider
- edifice.ProgressBar
- edifice.Dropdown
- Events
- Custom Widgets
- Hooks
- Styling
- Extra Elements
- Utility functions
- Developer Tools
- Release Notes
- Roadmap
Poetry Build System#
The Poetry pyproject.toml
specifies the package dependecies.
Because Edifice supports PySide6 and PyQt6 at the same time, neither
are required by [tool.poetry.dependencies]
. Instead they are both
optional [tool.poetry.group.dev.dependencies]
. A project which depends
on Edifice should also depend on either PySide6 or PyQt6.
The requirements.txt
is generated by
poetry export -f requirements.txt --output requirements.txt
License and Code Availability#
Edifice is released under the MIT License.
Edifice uses Qt under the hood, and both PyQt6 and PySide6 are supported. Note that PyQt6 is distributed with the GPL license while PySide6 is distributed under the more flexible LGPL license. See PyQt vs PySide Licensing.
The source code is avaliable on GitHub.
Support#
Submit bug reports or feature requests on Github Issues.
Submit any questions on Github Discussions.